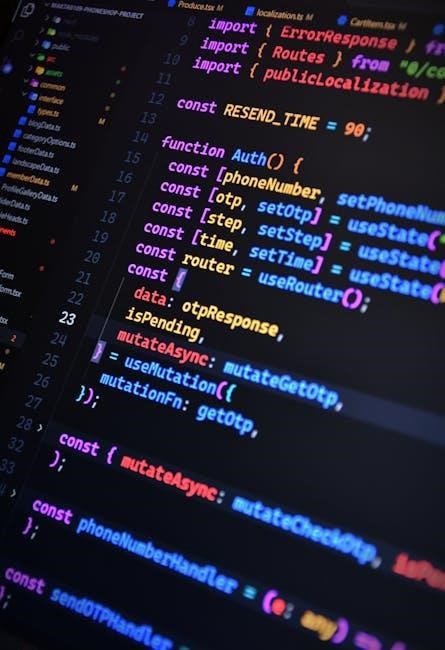
JavaScript and jQuery are essential tools for creating dynamic web applications․ JavaScript adds interactivity to websites‚ while jQuery simplifies complex tasks‚ making development faster and more efficient․
Overview of JavaScript and Its Role in Web Development
What is jQuery and Its Importance in Front-End Development
jQuery is a lightweight JavaScript library that simplifies front-end development by providing efficient tools for DOM manipulation‚ event handling‚ and animations․ It abstracts complex JavaScript operations into intuitive APIs‚ reducing development time and effort․ jQuery ensures cross-browser compatibility‚ allowing developers to write code that works seamlessly across different browsers․ Its extensive plugin ecosystem extends functionality‚ enabling tasks like form validation and AJAX requests․ By streamlining tasks‚ jQuery enhances productivity and code maintainability‚ making it a cornerstone for building dynamic‚ interactive web experiences efficiently․
Key Features of the Book “JavaScript and jQuery: Interactive Front-End Web Development”
JavaScript and jQuery: Interactive Front-End Web Development by Jon Duckett offers a comprehensive guide to modern web development․ The book focuses on practical‚ real-world examples‚ making complex concepts accessible․ It covers essential topics like DOM manipulation‚ event handling‚ and AJAX‚ with clear visuals and step-by-step explanations․ The visual learning approach helps readers grasp concepts quickly․ The book also emphasizes best practices for writing clean‚ maintainable code and optimizing performance․ By combining theory with hands-on exercises‚ it equips developers with the skills to build dynamic‚ user-friendly web applications․ This makes it an invaluable resource for both beginners and experienced developers․
Core Concepts of JavaScript
JavaScript Syntax and Basic Programming Concepts
JavaScript’s syntax is straightforward‚ resembling English‚ making it accessible for beginners․ It involves variables‚ data types like strings and numbers‚ and operators for manipulating values․ Conditional statements‚ such as if/else‚ control program flow‚ while loops (for‚ while) handle repetitive tasks․ Functions encapsulate reusable code‚ enhancing modularity․ Basic concepts like scope and hoisting are fundamental‚ ensuring variables behave predictably․ Proper indentation and semicolons are crucial for readable and error-free code․ Understanding these basics is essential for building dynamic web applications‚ as outlined in resources like Jon Duckett’s JavaScript and jQuery guide․
Working with Variables‚ Data Types‚ and Operators
In JavaScript‚ variables are declared using let‚ const‚ or var‚ storing values like strings‚ numbers‚ or booleans․ Data types include primitives (e․g․‚ string‚ number‚ boolean‚ null‚ undefined) and reference types like objects and arrays․ Operators perform actions: arithmetic (e․g․‚ +‚ *)‚ comparison (e․g․‚ ===‚ !=)‚ logical (e․g․‚ &&‚ ||)‚ and assignment (e․g․‚ =‚ +=)․ Understanding these elements is crucial for manipulating data and controlling program flow‚ as detailed in resources like Jon Duckett’s guide to JavaScript and jQuery․
Understanding Functions and Event Handling
Functions in JavaScript are reusable blocks of code that perform specific tasks‚ defined using the function keyword or arrow syntax․ They enhance code modularity and reusability․ Event handling involves responding to user interactions like clicks‚ key presses‚ or page loads․ JavaScript uses event listeners‚ attached via addEventListener‚ to trigger functions․ jQuery simplifies event handling with methods like ․click and ․on‚ making it easier to add interactivity․ Mastering functions and events is vital for creating dynamic‚ responsive web pages‚ as highlighted in Jon Duckett’s guide to JavaScript and jQuery for interactive front-end development․
jQuery simplifies JavaScript‚ aiding in DOM manipulation‚ event handling‚ and animations․ It enhances front-end development efficiency as detailed in Jon Duckett’s guide․
Getting Started with jQuery and Its Syntax
Selecting Elements and Manipulating the DOM
In jQuery‚ selecting elements is straightforward using CSS selectors․ For example‚ $('․class')
targets elements with a specific class‚ while $('#id')
selects an element by its ID․ Manipulating the DOM involves methods like ․css
to change styles‚ ․addClass
to add classes‚ and ․removeClass
to remove them․ You can also insert content using ․append
or ․prepend
․ jQuery simplifies dynamic updates‚ enabling real-time changes without reloading the page․ These techniques‚ as detailed in Jon Duckett’s guide‚ empower developers to create responsive and interactive web experiences efficiently‚ leveraging jQuery’s intuitive API for seamless DOM manipulation․ This foundation is crucial for building robust front-end applications․
Handling Events and Animations in jQuery
jQuery simplifies event handling with methods like ․click
‚ ․hover
‚ and ․submit
‚ allowing developers to attach functions to user interactions․ Animations are easily implemented using ․animate
‚ which can transition elements’ properties smoothly․ For example‚ $('․box')․animate({left: '250px'‚ opacity: '0․5'}‚ 1500);
moves and fades an element over 1․5 seconds․ Callback functions ensure actions execute in sequence‚ enhancing user experience․ These features‚ as covered in Jon Duckett’s guide‚ enable developers to create dynamic‚ engaging interfaces efficiently‚ making jQuery a powerful tool for front-end development․ By mastering events and animations‚ developers can build interactive web pages that captivate users and enhance functionality․
Practical Applications of JavaScript and jQuery
JavaScript and jQuery enable dynamic web interactions‚ enhancing user interfaces with animations‚ form validations‚ and real-time updates․ They power responsive designs‚ creating immersive digital experiences for users worldwide․
Building Interactive Web Pages and User Interfaces
JavaScript and jQuery are fundamental for crafting engaging web pages and user interfaces․ They enable dynamic interactions‚ such as form validations‚ animations‚ and responsive designs․ With jQuery‚ developers can effortlessly manipulate the DOM‚ handle events‚ and create visually appealing effects․ These tools empower the creation of intuitive interfaces that enhance user experience․ By integrating JavaScript and jQuery‚ developers can build modern‚ interactive web applications with features like real-time updates and dynamic content loading․ These capabilities are essential for delivering websites that captivate users and provide seamless functionality‚ making them indispensable in contemporary front-end development․
- Dynamic content manipulation
- Event-driven interactions
- Responsive design enhancements
- Real-time updates
Enhancing User Experience with Dynamic Content
Dynamism is key to captivating users‚ and JavaScript alongside jQuery excels in delivering this․ With JavaScript‚ you can dynamically update content without page reloads‚ enhancing user engagement․ jQuery simplifies DOM manipulation‚ enabling animations and transitions that create a polished interface․ Together‚ these tools allow developers to implement responsive designs‚ ensuring seamless experiences across devices․ Features like AJAX facilitate asynchronous data fetching‚ enabling real-time updates and reducing user wait times․ These techniques collectively contribute to a more intuitive and visually appealing web experience‚ keeping users engaged and improving overall satisfaction․
- Real-time content updates
- Animations and transitions
- Responsive design capabilities
- Asynchronous data loading
Real-World Examples of JavaScript and jQuery in Action
JavaScript and jQuery are widely used in real-world applications to create interactive and dynamic web experiences․ For instance‚ Yandex․Market leverages jQuery to implement B2B solutions‚ enhancing user interactions․ Similarly‚ Google Maps utilizes JavaScript to deliver real-time‚ interactive maps․ Social media platforms employ these technologies to create responsive feeds and instant updates․ E-commerce sites use JavaScript for shopping carts and jQuery for smooth animations‚ improving user engagement․ These examples highlight how JavaScript and jQuery are essential for building modern‚ interactive web applications that enhance user experience and drive business success․
- Yandex․Market’s B2B solutions
- Google Maps’ real-time interactivity
- Social media platforms’ dynamic feeds
- E-commerce shopping carts
Best Practices for Front-End Development
Adopting best practices ensures efficient and maintainable code․ Use clean syntax‚ optimize performance‚ and regularly test and debug to deliver robust web applications․
- Write clean‚ modular code
- Optimize for performance
- Test thoroughly
- Debug systematically
Writing Clean and Maintainable Code
Writing clean and maintainable code is crucial for efficient front-end development․ This involves using clear variable names‚ modular functions‚ and consistent indentation․ Avoid unnecessary complexity by breaking tasks into smaller‚ reusable functions․ Use comments to explain complex logic and ensure code readability․ Minimize dependencies and keep code organized to simplify future updates․ Regular code reviews and version control practices‚ like Git‚ help maintain code quality․ By following these practices‚ developers can create scalable‚ error-free applications that are easy to understand and modify‚ ensuring long-term project sustainability and collaboration․
Optimizing Performance in JavaScript Applications
Optimizing JavaScript performance involves reducing DOM manipulation‚ as it can cause layout recalculations and slow down rendering․ Minimizing heavy computations in loops and using requestAnimationFrame for animations can also enhance speed․ Avoiding unnecessary variables and optimizing event listeners by delegating events can prevent memory leaks and improve execution efficiency․ Additionally‚ leveraging browser caching and minimizing HTTP requests reduce load times․ Implementing lazy loading for images and code splitting for large applications further optimizes resource usage․ Profiling with browser DevTools helps identify bottlenecks‚ ensuring faster and smoother user experiences․
Debugging and Testing Your Code
Debugging and testing are crucial steps in ensuring robust JavaScript and jQuery applications․ Using browser developer tools like Chrome DevTools allows developers to inspect elements‚ monitor events‚ and debug code․ Techniques such as adding console․log statements or using breakpoints help identify issues․ Testing frameworks like Jasmine or QUnit enable automated unit testing‚ ensuring code reliability․ Additionally‚ version control systems like Git facilitate collaboration and rollback of changes․ Regular testing and debugging practices lead to cleaner‚ more maintainable code and improved user experiences․ By systematically identifying and resolving errors‚ developers can deliver high-quality web applications efficiently․